What Are APIs and How Can You Build One?
- Softude
- January 10, 2025
No application or software can work in isolation. They need APIs to work together and do what they are developed for. But what are APIs? What’s its role in software development? And how to build one? In this short guide, we will explain everything about APIs. Before that, understand why it is important for any application.

Applications with APIs are like well-connected hubs. They seamlessly integrate with other software and services. Let’s understand this with an example: Think of an e-commerce app that needs to connect with a digital wallet or online payment services to process transactions safely.
Without API, the application cannot process the transactions securely. To proceed, the developers must create custom code for encryption, bank communication, and compliance with financial regulations. This is time-consuming and unsafe, exposing the application to potential security vulnerabilities and compatibility issues.
On the other hand, the ecommerce app with API can securely authenticate the user and process the transactions in real time by connecting to external services like PayPal or Stripe. APIs, therefore, significantly enhance functionality, scalability, and user satisfaction while reducing implementation complexity.
What is an API and What Does it Do?
API is short for Application Programming Interface. Think of it like a window or bridge that allows your application to communicate with another. However, applications must follow certain rules and protocols to initiate communication with an external app or service. If the application gets a green signal, it can exchange data or request services from one another.
In simpler terms, APIs are similar to menus in a restaurant. The menu tells you what items you can order. In the software development world, these items are API endpoints, and the kitchen, which is the server, prepares what you request and sends it back.
How Do APIs Work?
APIs function by defining the communication rules between the requester, the client, and the responder, the server. Let’s see how communication happens between these two parties.
Step 1. Client Sends a Request
The client here is not an actual client but the application (like a mobile app or a website) that sends an API request. It is usually in the form of an HTTP (HyperText Transfer Protocol), which consists of the following:
- Endpoint: The URL specifies the requested resource (e.g., /users).
- Method: Defines the action, such as GET, POST, PUT, or DELETE.
- Headers: Metadata like authentication tokens or content type.
- Body (optional): Data sent with the request; typically, the data is in JSON format.
Step 2. The server Processes the Request
The request goes to the server, which gets the request, processes it, and interacts with a database or service to fulfill the request.
Step 3. Server Sends a Response
The client gets the response through the server against the request generated. The response contains data in JSON or XML format and a status code ( the code indicates whether the client gets the response or there is an error).
For example, imagine requesting user details from an API. The client makes a request, the server retrieves the information from its database, and the server responds with the requested data.
Types of APIs
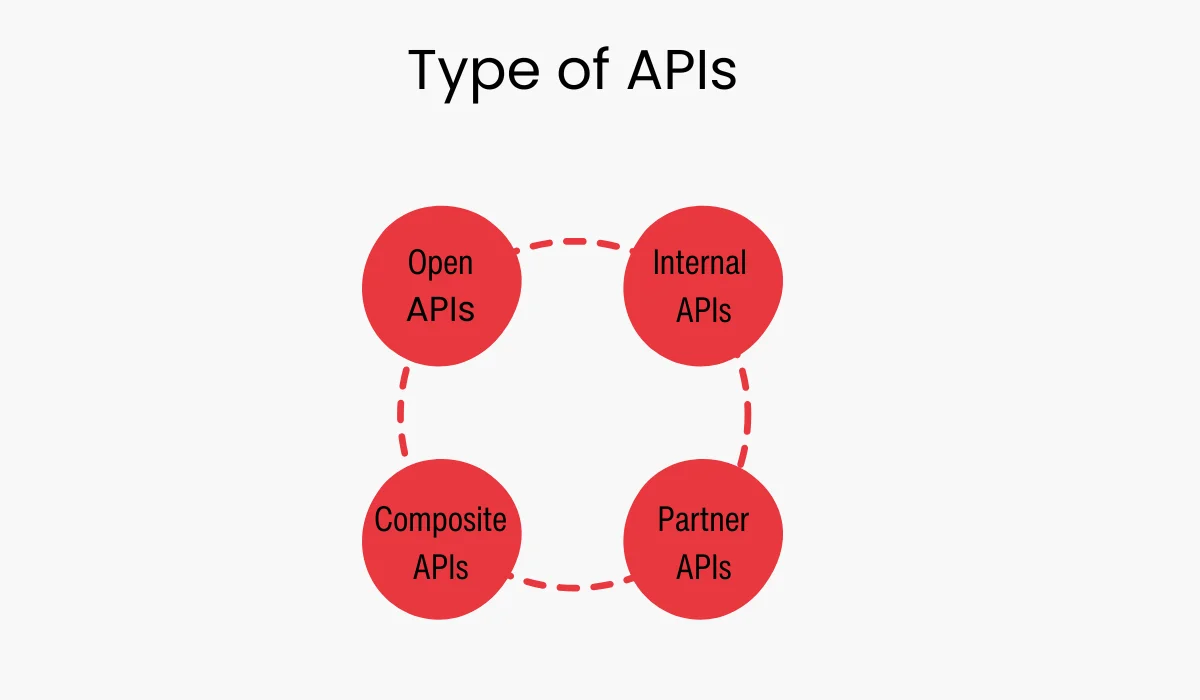
APIs come in various types, and though they all have the same purpose, they are used differently.
- Open APIs (Public APIs): These are available for public use, often to promote innovation (e.g., Twitter API, Google Maps API).
- Internal APIs: Such APIs are used within an organization to streamline internal processes.
- Partner APIs: They are used for collaboration and communication between specific business partners by sharing data.
- Composite APIs: These are groups of multiple API calls into one to reduce network overhead.
Also Read: REST API vs RESTful API: Which is Good for Web App Development?
What is API Development?
API development is the process of creating a set of rules, protocols, and tools that enable different software applications to communicate and interact with each other. There are a few key components for developing APIs.
- API Design: First, define the structure of the API. The structure has endpoints, methods (GET, POST, PUT, DELETE), request/response formats, and data models.
- API Implementation: Write the backend code for APIs to perform the desired functions and process the requests. Programming languages like Python, Node.js, Java, or PHP are used here.
- Security: Implement authentication (e.g., OAuth, API keys) and authorization mechanisms to ensure only authorized users or systems can access the API.
- Testing: Test your APIs for security, functionality, and performance.
- Deployment: Host the API on a server or cloud platform (e.g., AWS, Azure, Google Cloud) so that it can be accessed over the internet. Docker and Kubernetes are used for scalability and containerization.
Tools to Use for API Development
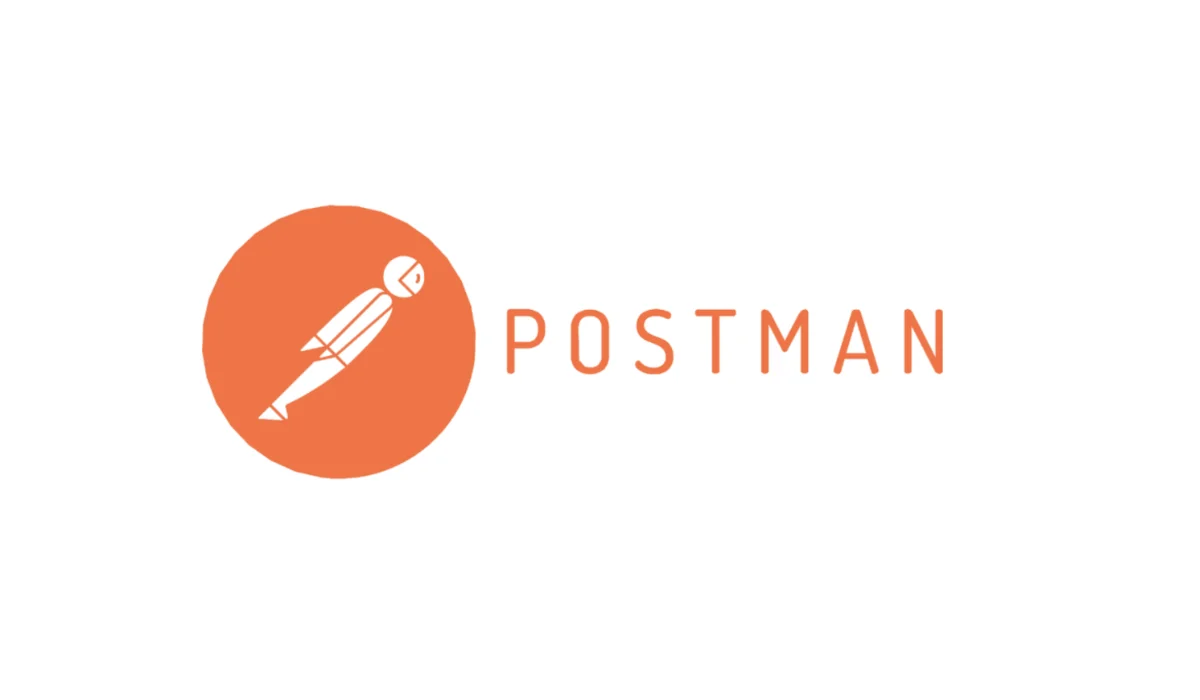
If you want to learn API integration and development, you must be familiar with the popular API tools.
1. Postman
Postman is one of the most powerful API development tools. More than 35 million developers use this platform to design, test, and document APIs. Developers can even share custom-built APIs for unique application functionality.
2. Swagger (OpenAPI)
Swagger is a framework publicly available for developers worldwide for designing and documenting APIs at scale. It provides interactive documentation that allows users to test endpoints directly from the documentation. Swagger is the best tool for beginners as it provides all the learning resources to build an API from scratch.
3. Insomnia
If Postman seems expensive or complicated, Insomnia is the best lightweight alternative. It is great for testing APIs and handling complex workflows.
- RESTful API Libraries and Frameworks
- Node.js + Express: Ideal for building RESTful APIs in JavaScript.
- Python + Flask/Django: Popular for rapid API development.
- Ruby on Rails: Great for building APIs in Ruby.
- Spring Boot: A robust framework for creating APIs in Java.
4. Version Control Tools
Git, GitHub, or GitLab are the must-have tools in your API development tech stack. These tools ensure the proper versioning of your API’s source code and help developers to collaborate.
Also Read: APIs Vs Microservices: 4 Key Differences You Did Not Know Before
9 Best Practices for API Development
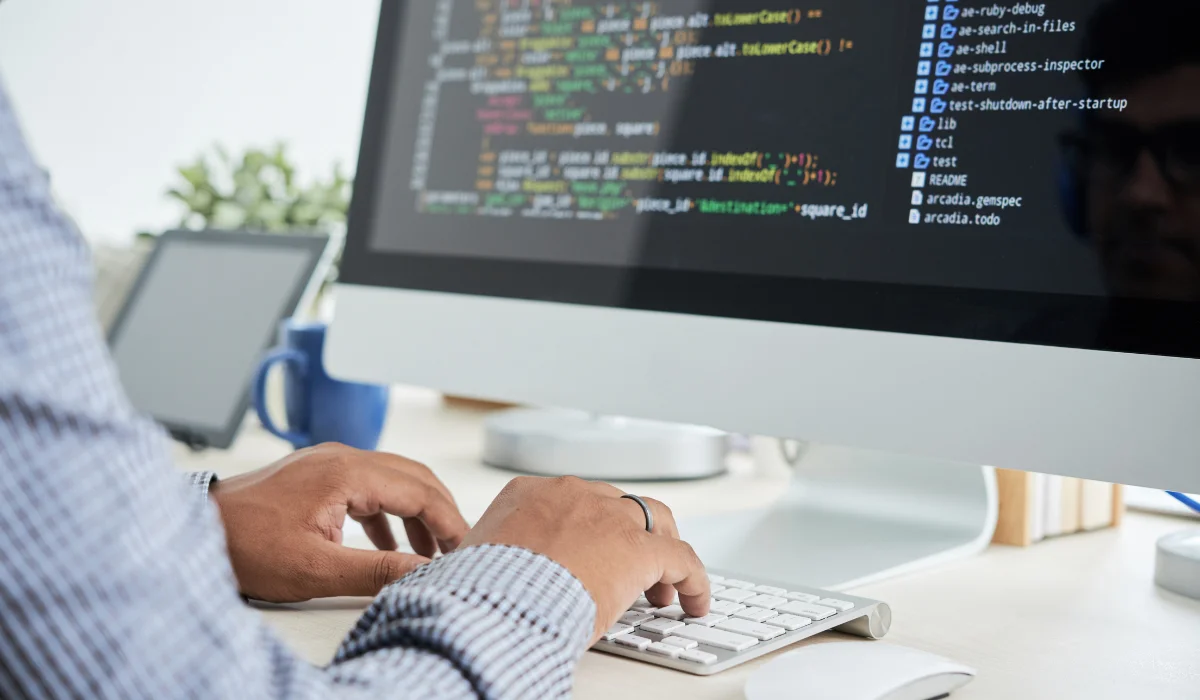
Despite having the best API tools, your code may fail if you don’t know these best practices. After all, developing APIs involves more than just writing code.
1. Design First, Code Later
Instead of writing code directly, start by designing the API. Use tools like Swagger or API Blueprint to do this. They clarify what the API should do before you start coding.
2. Use RESTful Principles
Adopt REST (Representational State Transfer) principles for simplicity and scalability. Use clear, descriptive endpoints (e.g., /users/products). Rely on HTTP methods (GET for retrieval, POST for creation, etc.). Use proper status codes (e.g., 200 for success, 404 for not found).
3. Implement Authentication and Authorization
Secure your API with OAuth 2.0, a popular authorization framework. Also, use JWT (JSON Web Tokens) for stateless authentication. API Keys help in basic use cases.
- Validate and Sanitize Inputs
- Prevent security vulnerabilities like SQL injection or XSS by validating user inputs.
4. Paginate Responses
Implement pagination for large datasets to improve performance and reduce bandwidth usage. For example, when fetching data for many users, only fetch a specific number at a time, such as 50 users per page.
5. Use Versioning
Versioning your API ensures backward compatibility. For example, having /v1/ and /v2/ versions of the same API helps manage changes without breaking existing systems.
6. Write Clear Documentation
Comprehensive documentation is vital. Include examples, error codes, and usage scenarios. Tools like Swagger can generate interactive docs.
7. Test Thoroughly
Use tools like Postman, Insomnia, or automated testing frameworks to:
- Test endpoints for expected responses.
- Simulate edge cases and error scenarios.
- Monitor performance under load.
8. Log and Monitor Usage
Implement logging for API requests and errors. Monitoring tools like New Relic or Datadog can be used to track performance and detect issues.
9. Optimize Performance
Use caching (e.g., Redis) to reduce redundant database queries.Minimize payload size by sending only necessary data and optimize database queries to prevent bottlenecks.
Conclusion
Like frameworks or other important technologies, APIs are the backbone of applications and modern software systems. Understanding APIs, how they work, and how to build them effectively ensures these backbones are well-developed. Also, the principles, tools, and best practices outlined in this guide are must-knows for API development.
Liked what you read?
Subscribe to our newsletter